Automatic Watering in Plant
Heading 1
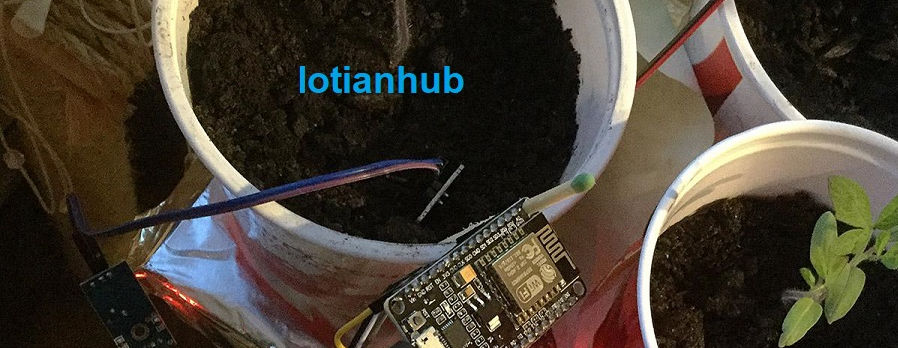
It usually consists of a central microcontroller to which other objects are connected. The smart garden consists of NodeMCU as a hub to which different types of sensors such as moisture sensor, humidity sensor, temperature sensor and ultrasonic sensor are connected. The ultrasonic sensor is connected to a water tank which indicated the level of water in the tank. Other sensors are connected to their respective positions and these sensors send the data to NodeMCU which consists of an inbuilt Wi-Fi technology. Firebase is a database available on the internet in which real-time values of the sensor are updated every second. Android application is developed using android studio software. Within the software, the connectivity between the application and firebase will be made. So, the user can monitor the parameters from anywhere. Watering of garden varies with the type of soil. Hence the values of the sensors are predetermined for automation purposes inside the software. Whenever the user finds need of watering the garden, a switch in the application will automate the process. This helps in complete maintenance of the garden.
Components:
- NodeMCU ESP8266
- Soil Moisture Sensor Module
- Water Pump Module
- Relay Module
- DHT11
Code:
#include <DHT.h>
#include <ESP8266WiFi.h>
String apiKey = "X5AQ3EGIKMBYW31H"; // Enter your Write API key here
const char* server = "api.thingspeak.com";
const char *ssid = "CircuitLoop"; // Enter your WiFi Name
const char *pass = "circuitdigest101"; // Enter your WiFi Password
#define DHTPIN D3 // GPIO Pin where the dht11 is connected
DHT dht(DHTPIN, DHT11);
WiFiClient client;
const int moisturePin = A0; // moisteure sensor pin
const int motorPin = D0;
unsigned long interval = 10000;
unsigned long previousMillis = 0;
unsigned long interval1 = 1000;
unsigned long previousMillis1 = 0;
float moisturePercentage; //moisture reading
float h; // humidity reading
float t; //temperature reading
void setup()
{
Serial.begin(115200);
delay(10);
pinMode(motorPin, OUTPUT);
digitalWrite(motorPin, LOW); // keep motor off initally
dht.begin();
Serial.println("Connecting to ");
Serial.println(ssid);
WiFi.begin(ssid, pass);
while (WiFi.status() != WL_CONNECTED)
{
delay(500);
Serial.print("."); // print ... till not connected
}
Serial.println("");
Serial.println("WiFi connected");
}
void loop()
{
unsigned long currentMillis = millis(); // grab current time
h = dht.readHumidity(); // read humiduty
t = dht.readTemperature(); // read temperature
if (isnan(h) || isnan(t))
{
Serial.println("Failed to read from DHT sensor!");
return;
}
moisturePercentage = ( 100.00 - ( (analogRead(moisturePin) / 1023.00) * 100.00 ) );
if ((unsigned long)(currentMillis - previousMillis1) >= interval1) {
Serial.print("Soil Moisture is = ");
Serial.print(moisturePercentage);
Serial.println("%");
previousMillis1 = millis();
}
if (moisturePercentage < 50) {
digitalWrite(motorPin, HIGH); // tun on motor
}
if (moisturePercentage > 50 && moisturePercentage < 55) {
digitalWrite(motorPin, HIGH); //turn on motor pump
}
if (moisturePercentage > 56) {
digitalWrite(motorPin, LOW); // turn off mottor
}
if ((unsigned long)(currentMillis - previousMillis) >= interval) {
sendThingspeak(); //send data to thing speak
previousMillis = millis();
client.stop();
}
}
void sendThingspeak() {
if (client.connect(server, 80))
{
String postStr = apiKey; // add api key in the postStr string
postStr += "&field1=";
postStr += String(moisturePercentage); // add mositure readin
postStr += "&field2=";
postStr += String(t); // add tempr readin
postStr += "&field3=";
postStr += String(h); // add humidity readin
postStr += "\r\n\r\n";
client.print("POST /update HTTP/1.1\n");
client.print("Host: api.thingspeak.com\n");
client.print("Connection: close\n");
client.print("X-THINGSPEAKAPIKEY: " + apiKey + "\n");
client.print("Content-Type: application/x-www-form-urlencoded\n");
client.print("Content-Length: ");
client.print(postStr.length()); //send lenght of the string
client.print("\n\n");
client.print(postStr); // send complete string
Serial.print("Moisture Percentage: ");
Serial.print(moisturePercentage);
Serial.print("%. Temperature: ");
Serial.print(t);
Serial.print(" C, Humidity: ");
Serial.print(h);
Serial.println("%. Sent to Thingspeak.");
}
}
Conclusions:
The Smart irrigation System has wide scope to automate the complete irrigation system. Here we are building a IoT based Irrigation System using ESP8266 NodeMCU Module and DHT11 Sensor. It will not only automatically irrigate the water based on the moisture level in the soil but also send the Data to ThingSpeak Server to keep track of the land condition. The System will consist a water pump which will be used to sprinkle water on the land depending upon the land environmental condition such as Moisture, Temperature and Humidity.